In the world of programming, Object-Oriented Programming (OOP) is a powerful concept that helps developers organize and structure their code in a more logical and efficient way. Instead of treating programs as a series of instructions to be executed sequentially, OOP allows us to model real-world entities as objects with properties and behaviors. Let’s dive into the basics of OOP and explore how it’s used in PHP.
Object-Oriented Programming (OOP) Explained
Object-Oriented Programming, or OOP for short, revolves around the concept of objects. But what exactly is an object? Well, think of an object as a self-contained unit that combines data (known as properties or attributes) and functions (known as methods) that operate on that data. These objects can interact with each other, exchanging messages and collaborating to achieve specific tasks.
The core principles of OOP include:
- Encapsulation: Encapsulation is like putting an object in a box and allowing access only through predefined openings. It hides the internal state of an object and only exposes the necessary functionality through well-defined interfaces. This promotes data abstraction and helps in managing complexity.
- Inheritance: Inheritance allows objects to inherit properties and behaviors from other objects. This promotes code reusability and helps in creating hierarchical relationships between objects.
- Polymorphism: Polymorphism allows objects of different types to be treated as objects of a common superclass. It enables a single interface to represent multiple underlying data types, making the code more flexible and adaptable.
Commonly Asked Questions about OOP in PHP
- Why do we use
$this->name
instead of$this->$name
?
- The
$this->name
syntax is used to access the propertyname
of the current object within a class in PHP. The->
arrow operator is used to access properties and methods of an object. On the other hand,$this->$name
would be interpreted as trying to access the property whose name is stored in the variable$name
, which is not the intended behavior.
- What is the significance of access modifiers like public, private, and protected?
- Access modifiers control the visibility of properties and methods within a class.
public
: Properties and methods declared as public can be accessed from outside the class.private
: Properties and methods declared as private can only be accessed within the class itself.protected
: Properties and methods declared as protected can be accessed within the class itself and its subclasses.
Example: Understanding OOP with PHP
<?php
class Animal {
protected $name;
public function __construct($name) {
$this->name = $name;
}
public function speak() {
echo "{$this->name} says hello!";
}
}
class Dog extends Animal {
public function speak() {
echo "{$this->name} barks!";
}
}
class Cat extends Animal {
public function speak() {
echo "{$this->name} meows!";
}
}
// Create objects of Dog and Cat classes
$dog = new Dog("Buddy");
$cat = new Cat("Whiskers");
// Call the speak method for each object
$dog->speak(); // Output: Buddy barks!
$cat->speak(); // Output: Whiskers meows!
?>
In this example, we have a base class Animal
with a property $name
and a method speak()
. We then create two subclasses, Dog
and Cat
, which inherit from the Animal
class. Each subclass overrides the speak()
method to provide a specific implementation. Finally, we create objects of the Dog
and Cat
classes and call the speak()
method for each object, demonstrating polymorphism in action.
Let’s break down the code flow of the provided example step by step:
Class Definitions:
- We define three classes:
Animal
,Dog
, andCat
. - Each class represents a different type of animal.
- The
Animal
class has a property$name
and a methodspeak()
. - The
Dog
andCat
classes inherit from theAnimal
class.
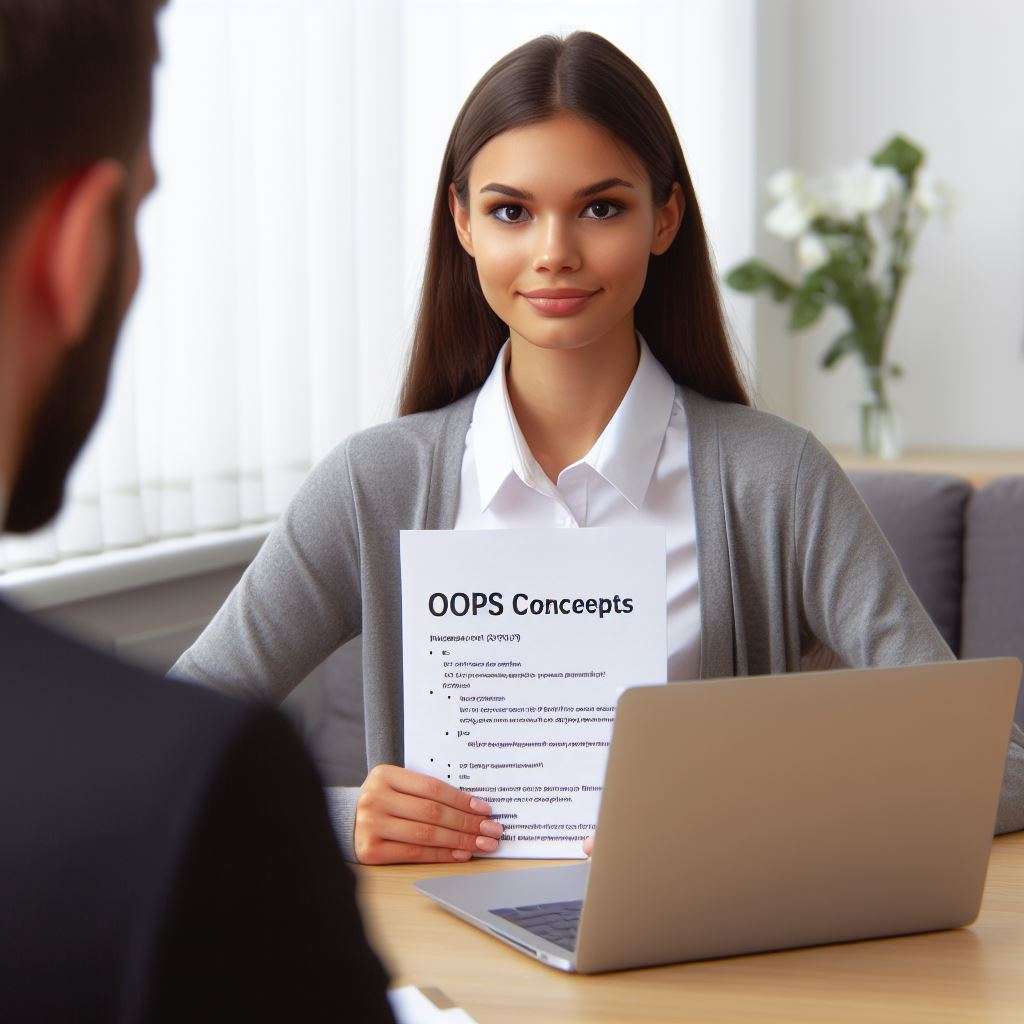
Object Instantiation:
- We create two objects:
$dog
of theDog
class and$cat
of theCat
class. - For each object, we provide a name (“Buddy” for the dog and “Whiskers” for the cat) to the constructor.
Method Invocation:
- We call the
speak()
method for each object ($dog->speak()
and$cat->speak()
). - When the
speak()
method is called, PHP looks for the method in the class of the object being referenced. If it’s not found, it traverses up the inheritance hierarchy until it finds the method.
Method Execution:
- For the
$dog
object, thespeak()
method from theDog
class is executed. It outputs “Buddy barks!”. - For the
$cat
object, thespeak()
method from theCat
class is executed. It outputs “Whiskers meows!”.
Output:
- Finally, the outputs “Buddy barks!” and “Whiskers meows!” are displayed on the screen.
So, the code flow can be summarized as follows:
- Create an object of the
Dog
class with the name “Buddy”. - Call the
speak()
method for theDog
object. - Execute the
speak()
method from theDog
class, which outputs “Buddy barks!”. - Create an object of the
Cat
class with the name “Whiskers”. - Call the
speak()
method for theCat
object. - Execute the
speak()
method from theCat
class, which outputs “Whiskers meows!”.
This sequence of steps demonstrates how inheritance and method overriding work in PHP, allowing objects of different classes to exhibit polymorphic behavior.
In summary, Object-Oriented Programming is a powerful paradigm that allows us to model real-world entities as objects with properties and behaviors. By understanding the core principles of OOP and applying them effectively in PHP, we can write more organized, modular, and maintainable code.