Introduction:
Exception handling and structured data formatting, particularly in JSON, are fundamental aspects of modern programming. Java and Python, two widely used programming languages, offer different tools and methods to manage errors and structure data effectively. In this blog post, we’ll explore common error scenarios programmers encounter in JSON formatting and exception handling within Java and Python. We’ll delve into solutions for these issues, utilizing keywords like “Exception Handling,” “JSON Formatting,” “Java Programming,” “Python Programming,” and more. By understanding and resolving these challenges, programmers can enhance their code quality and develop more resilient applications.
1. Exception Handling in JSON Format
Java:
Challenge: Constructing a structured JSON representation of an exception in Java.
Solution:
try {
// Code that may throw an exception
} catch (Exception e) {
String exceptionJson = "{\"exception\": \"" + e.getClass().getSimpleName() + "\", \"message\": \"" + e.getMessage() + "\"}";
System.out.println(exceptionJson);
}
Python:
Challenge: Formatting an exception as JSON in Python.
Solution:
import json
try:
# Code that may raise an exception
except Exception as e:
exception_json = json.dumps({"exception": type(e).__name__, "message": str(e)})
print(exception_json)
2. Common JSON Formatting Mistakes
Challenge: Incorrect JSON Format Creation
Solution – Java (using Gson):
import com.google.gson.Gson;
class ExampleObject {
public String name;
public int age;
}
public class JsonHandling {
public static void main(String[] args) {
ExampleObject obj = new ExampleObject();
obj.name = "Alice";
obj.age = 25;
Gson gson = new Gson();
String json = gson.toJson(obj);
System.out.println(json);
}
}
Solution – Python:
import json
data = {
"name": "Alice",
"age": 25
}
json_data = json.dumps(data)
print(json_data)
3. Parsing JSON Data
Challenge: Parsing JSON with Syntax Errors
Solution – Java (using Jackson):
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonParsing {
public static void main(String[] args) {
String invalidJson = "{'name': 'John', 'age': 30}"; // Incorrect JSON format
ObjectMapper objectMapper = new ObjectMapper();
try {
// Handling exception while parsing
objectMapper.readTree(invalidJson);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Solution – Python:
import json
invalid_json = "{'name': 'John', 'age': 30}" # Incorrect JSON format
try:
# Handling exception while parsing
json.loads(invalid_json)
except json.JSONDecodeError as e:
print(e)
4. Invalid JSON Structure
Challenge: Dealing with Invalid JSON Structures
Solution – Java (using Gson):
import com.google.gson.JsonSyntaxException;
public class JsonStructure {
public static void main(String[] args) {
String invalidJson = "{\"name\": \"John\", \"age\": 30"; // Missing closing brace
try {
new Gson().fromJson(invalidJson, Object.class);
} catch (JsonSyntaxException e) {
e.printStackTrace();
}
}
}
Solution – Python:
import json
invalid_json = '{"name": "John", "age": 30' # Missing closing brace
try:
json.loads(invalid_json)
except json.JSONDecodeError as e:
print(e)
5. Encoding and Decoding Errors
Challenge: Encoding and Decoding Issues in JSON
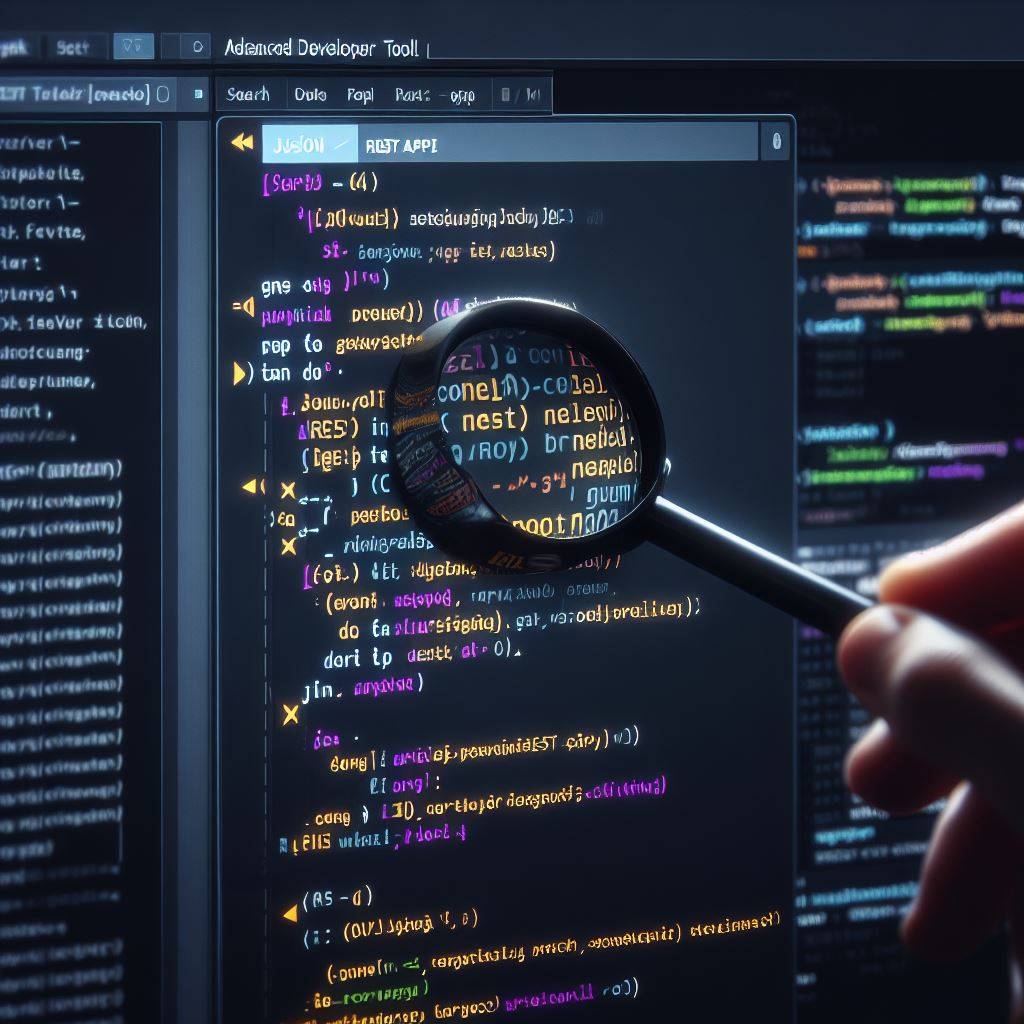
Solution – Java (using Jackson):
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonEncoding {
public static void main(String[] args) {
ObjectMapper mapper = new ObjectMapper();
try {
// Encoding issue example
String json = mapper.writeValueAsString(new Object());
} catch (JsonProcessingException e) {
e.printStackTrace();
}
}
}
Solution – Python:
import json
try:
# Decoding issue example
json.loads("This is not a valid JSON")
except json.JSONDecodeError as e:
print(e)
Mastering these error scenarios not only improves code quality but also equips developers with the necessary skills to handle exceptions and JSON formatting effectively in both Java and Python.
Conclusion:
In the world of software development, mastering the art of exception handling and crafting well-structured JSON data is pivotal. Through the solutions provided for various error scenarios in both Java and Python, developers gain a deeper understanding of “Error Scenarios,” “Error Handling,” and “Structured Data Handling.” These essential skills not only bolster the robustness of code but also equip programmers with the necessary tools for effective “Debugging Techniques.” As they navigate through challenges and find resolutions, their ability to handle exceptions and format JSON data efficiently becomes a valuable asset in their programming toolkit.