Introduction:
JavaScript Errors, such as the “TypeError: Cannot read property ‘X’ of undefined” and the need for default values in object destructuring, can be perplexing. This guide will decode these JavaScript Errors, providing practical solutions. By the end, you’ll confidently navigate and resolve these common challenges, enhancing your JavaScript development skills. Join us on this journey through the world of JavaScript Errors.
How to Assign Default Values in Object Destructuring in JavaScript?
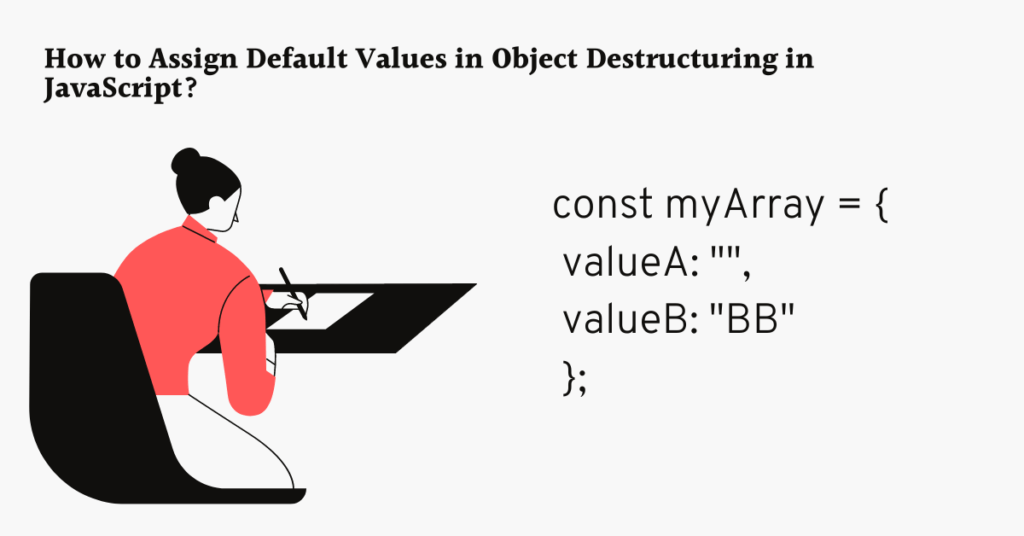
In JavaScript, object destructuring allows you to extract values from objects easily. But what if you want to set default values for these extracted values? Let’s explore how to do that.
Suppose you have an object like this:
const myArray = {
valueA: "",
valueB: "BB"
};
And you want to assign default values during destructuring. For instance, you want to give “E” as the default value for ‘valueA
‘ if it’s empty or undefined.
// Define the object
const myArray = {
valueA: "",
valueB: "BB"
};
// Use object destructuring with default values
let { valueA: A = "E", valueB: B } = myArray;
console.log(A); // "E" (default value assigned)
console.log(B); // "BB"
In this example, we’re using object destructuring to extract valueA
and valueB
from myArray
. We assign a default value of “E” to A
using = "E"
. If valueA
is empty or undefined, A
gets the default value “E.” On the other hand, B
retains its original value from myArray
.
TypeError: Cannot read property ‘X’ of undefined”
Error Explanation:
You get this error in JavaScript when you try to read a property (let’s call it ‘X’) from an object, but the object doesn’t exist or has no value. In plain terms, it’s like trying to find something in nothing.
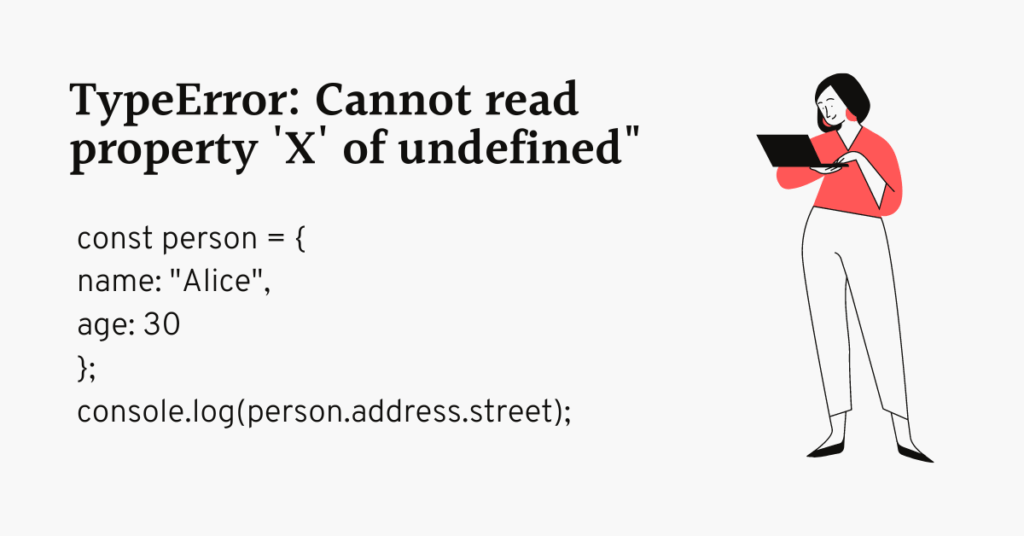
Common Causes:
Here’s why this error usually happens:
- Undefined Object: You forgot to create or set up the object before trying to use it.
- Null Object: You intentionally made the object empty, like having an empty box.
- Mistyped or Missing Property: Sometimes, you think ‘X’ is there, but it’s not; it’s like searching for a hidden treasure that isn’t hidden anywhere.
Example:
- Let’s see it in action:
// We have some information about a person
const person = {
name: "Alice",
age: 30
};
// Now, we're trying to find a piece of info that doesn't exist
console.log(person.address.street);
In this example, we’re looking for the ‘street’ address of a person, but we never actually told JavaScript about it. So, it’s like trying to find a secret path on a map that isn’t there.
Solution:
To avoid this error, just remember to:
- Make sure the object is there and ready before using it.
- Double-check that the ‘X’ property you’re searching for actually exists.
- You can also use a modern trick (optional chaining) to search safely:
console.log(person.address?.street); // No error, it's like saying "find it if it's there"
By following these steps, you can steer clear of the “TypeError: Cannot read property ‘X’ of undefined” error in your JavaScript code.